2 Introduction
You can label chapter and section titles using {#label}
after them, e.g., we can reference Chapter 2. If you do not manually label them, there will be automatic labels anyway, e.g., Chapter 4.
Figures and tables with captions will be placed in figure
and table
environments, respectively.
par(mar = c(4, 4, .1, .1))
plot(pressure, type = 'b', pch = 19)
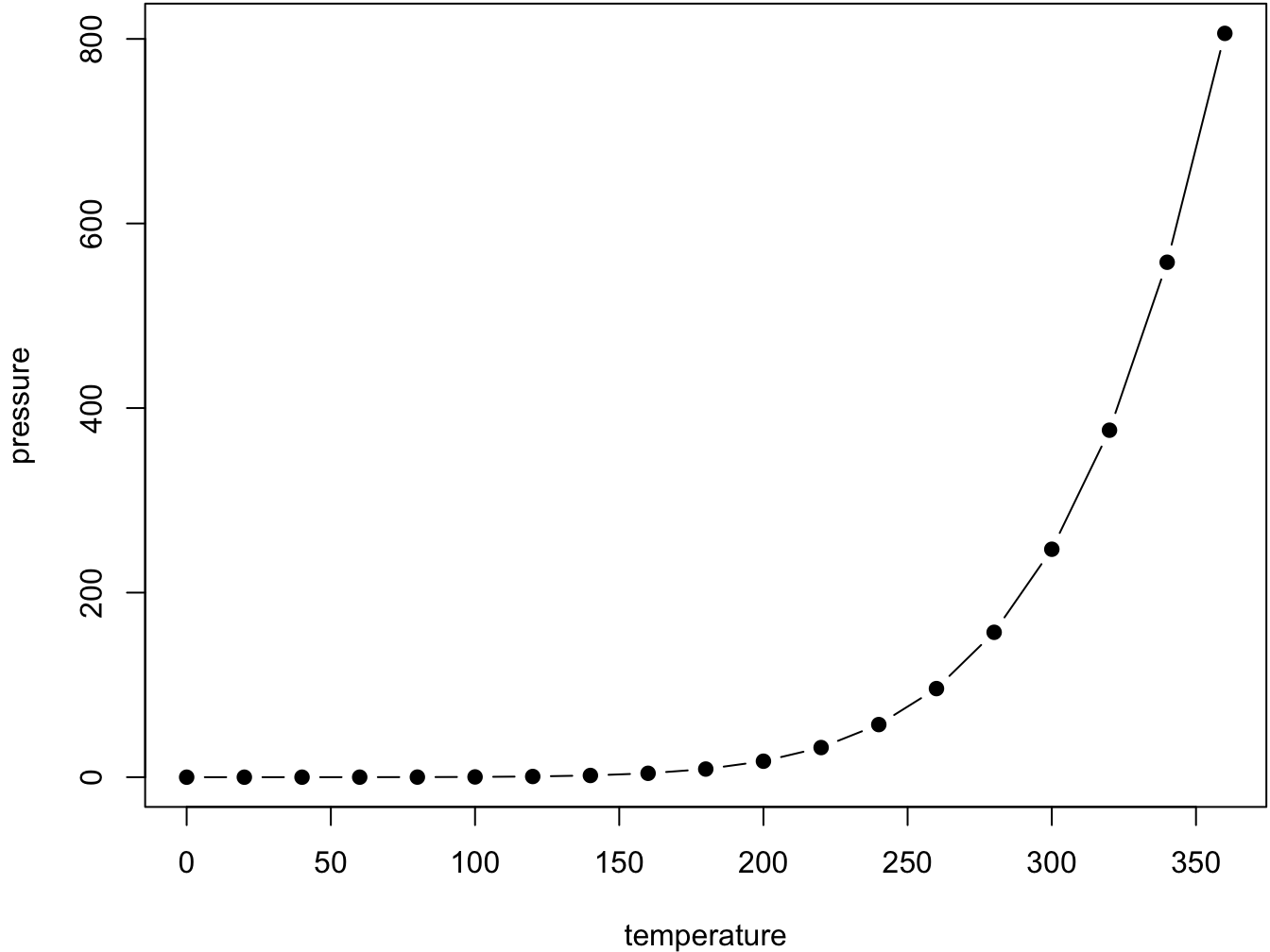
Figure 2.1: Here is a nice figure!
Reference a figure by its code chunk label with the fig:
prefix, e.g., see Figure 2.1. Similarly, you can reference tables generated from knitr::kable()
, e.g., see Table 2.1.
::kable(
knitrhead(iris, 20), caption = 'Here is a nice table!',
booktabs = TRUE
)
Sepal.Length | Sepal.Width | Petal.Length | Petal.Width | Species |
---|---|---|---|---|
5.1 | 3.5 | 1.4 | 0.2 | setosa |
4.9 | 3.0 | 1.4 | 0.2 | setosa |
4.7 | 3.2 | 1.3 | 0.2 | setosa |
4.6 | 3.1 | 1.5 | 0.2 | setosa |
5.0 | 3.6 | 1.4 | 0.2 | setosa |
5.4 | 3.9 | 1.7 | 0.4 | setosa |
4.6 | 3.4 | 1.4 | 0.3 | setosa |
5.0 | 3.4 | 1.5 | 0.2 | setosa |
4.4 | 2.9 | 1.4 | 0.2 | setosa |
4.9 | 3.1 | 1.5 | 0.1 | setosa |
5.4 | 3.7 | 1.5 | 0.2 | setosa |
4.8 | 3.4 | 1.6 | 0.2 | setosa |
4.8 | 3.0 | 1.4 | 0.1 | setosa |
4.3 | 3.0 | 1.1 | 0.1 | setosa |
5.8 | 4.0 | 1.2 | 0.2 | setosa |
5.7 | 4.4 | 1.5 | 0.4 | setosa |
5.4 | 3.9 | 1.3 | 0.4 | setosa |
5.1 | 3.5 | 1.4 | 0.3 | setosa |
5.7 | 3.8 | 1.7 | 0.3 | setosa |
5.1 | 3.8 | 1.5 | 0.3 | setosa |
You can write citations, too. For example, we are using the bookdown package (Xie 2020) in this sample book, which was built on top of R Markdown and knitr (Xie 2015).
2.1 Here adding new test
library(pins)
board_register(name = "pins_board", url = "https://raw.githubusercontent.com/predictcrypto/pins/master/", board = "datatxt")
pin_get(name = "hitBTC_orderbook") cryptodata <-
Show data
cryptodata
## # A tibble: 244,443 x 27
## pair symbol quote_currency ask_1_price ask_1_quantity ask_2_price
## <chr> <chr> <chr> <dbl> <dbl> <dbl>
## 1 BTCU… BTC USD 16290. 0.0589 16290.
## 2 ETHU… ETH USD 462. 0.4 463.
## 3 EOSU… EOS USD 2.46 600 2.46
## 4 LTCU… LTC USD 60.7 3.75 60.7
## 5 BSVU… BSV USD 158. 0.6 158.
## 6 ADAU… ADA USD 0.105 775 0.105
## 7 ZECU… ZEC USD 63.0 2.59 63.1
## 8 TRXU… TRX USD 0.0249 65497 0.0249
## 9 HTUSD HT USD 3.64 105. 3.65
## 10 XMRU… XMR USD 112. 0.023 112.
## # … with 244,433 more rows, and 21 more variables: ask_2_quantity <dbl>,
## # ask_3_price <dbl>, ask_3_quantity <dbl>, ask_4_price <dbl>,
## # ask_4_quantity <dbl>, ask_5_price <dbl>, ask_5_quantity <dbl>,
## # bid_1_price <dbl>, bid_1_quantity <dbl>, bid_2_price <dbl>,
## # bid_2_quantity <dbl>, bid_3_price <dbl>, bid_3_quantity <dbl>,
## # bid_4_price <dbl>, bid_4_quantity <dbl>, bid_5_price <dbl>,
## # bid_5_quantity <dbl>, date_time_utc <dttm>, date <date>, pkDummy <chr>,
## # pkey <chr>
Show nested data
library(tidyverse)
## ── Attaching packages ─────────────────────────────────────── tidyverse 1.3.0 ──
## ✔ ggplot2 3.3.2 ✔ purrr 0.3.4
## ✔ tibble 3.0.4 ✔ dplyr 1.0.2
## ✔ tidyr 1.1.2 ✔ stringr 1.4.0
## ✔ readr 1.4.0 ✔ forcats 0.5.0
## ── Conflicts ────────────────────────────────────────── tidyverse_conflicts() ──
## ✖ dplyr::filter() masks stats::filter()
## ✖ dplyr::lag() masks stats::lag()
group_by(cryptodata, symbol)
cryptodata <-nest(cryptodata)
## # A tibble: 218 x 2
## # Groups: symbol [218]
## symbol data
## <chr> <list>
## 1 BTC <tibble [2,292 × 26]>
## 2 ETH <tibble [1,416 × 26]>
## 3 EOS <tibble [2,245 × 26]>
## 4 LTC <tibble [2,292 × 26]>
## 5 BSV <tibble [1,535 × 26]>
## 6 ADA <tibble [1,178 × 26]>
## 7 ZEC <tibble [1,133 × 26]>
## 8 TRX <tibble [1,513 × 26]>
## 9 HT <tibble [2,227 × 26]>
## 10 XMR <tibble [2,286 × 26]>
## # … with 208 more rows
What does DT look like
library(DT)
cryptodata
## # A tibble: 244,443 x 27
## # Groups: symbol [218]
## pair symbol quote_currency ask_1_price ask_1_quantity ask_2_price
## <chr> <chr> <chr> <dbl> <dbl> <dbl>
## 1 BTCU… BTC USD 16290. 0.0589 16290.
## 2 ETHU… ETH USD 462. 0.4 463.
## 3 EOSU… EOS USD 2.46 600 2.46
## 4 LTCU… LTC USD 60.7 3.75 60.7
## 5 BSVU… BSV USD 158. 0.6 158.
## 6 ADAU… ADA USD 0.105 775 0.105
## 7 ZECU… ZEC USD 63.0 2.59 63.1
## 8 TRXU… TRX USD 0.0249 65497 0.0249
## 9 HTUSD HT USD 3.64 105. 3.65
## 10 XMRU… XMR USD 112. 0.023 112.
## # … with 244,433 more rows, and 21 more variables: ask_2_quantity <dbl>,
## # ask_3_price <dbl>, ask_3_quantity <dbl>, ask_4_price <dbl>,
## # ask_4_quantity <dbl>, ask_5_price <dbl>, ask_5_quantity <dbl>,
## # bid_1_price <dbl>, bid_1_quantity <dbl>, bid_2_price <dbl>,
## # bid_2_quantity <dbl>, bid_3_price <dbl>, bid_3_quantity <dbl>,
## # bid_4_price <dbl>, bid_4_quantity <dbl>, bid_5_price <dbl>,
## # bid_5_quantity <dbl>, date_time_utc <dttm>, date <date>, pkDummy <chr>,
## # pkey <chr>
And nested
nest(cryptodata)
## # A tibble: 218 x 2
## # Groups: symbol [218]
## symbol data
## <chr> <list>
## 1 BTC <tibble [2,292 × 26]>
## 2 ETH <tibble [1,416 × 26]>
## 3 EOS <tibble [2,245 × 26]>
## 4 LTC <tibble [2,292 × 26]>
## 5 BSV <tibble [1,535 × 26]>
## 6 ADA <tibble [1,178 × 26]>
## 7 ZEC <tibble [1,133 × 26]>
## 8 TRX <tibble [1,513 × 26]>
## 9 HT <tibble [2,227 × 26]>
## 10 XMR <tibble [2,286 × 26]>
## # … with 208 more rows
References
Xie, Yihui. 2015. Dynamic Documents with R and Knitr. 2nd ed. Boca Raton, Florida: Chapman; Hall/CRC. http://yihui.name/knitr/.
Xie, Yihui. 2020. Bookdown: Authoring Books and Technical Documents with R Markdown. https://github.com/rstudio/bookdown.